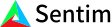
- Bob Anderson
- Sign In

Sentim's Emotion APIs v1.0.0
Scroll down for code samples, example requests and responses. Select a language for code samples from the tabs above or the mobile navigation menu.
An emotion recognition api that tells you the emotion of text, and not just the connotation.
Getting Started: follow the instructions under First Time Authentication, and then use one of the code examples provided.
Python API Client:
Base URLs:
Terms of service Email: Support
Authentication
-
oAuth2 authentication.
-
Flow: clientCredentials
-
First Time Authentication:
1. Go to https://sentimllc.com/user.html
2. Log in (or create an account if you haven't made one yet)
3. Click on the "Generate" button in your User settings to generate a file containing your client ID and secret
4. Store the generated file in a safe, non-public location
5. Call get_access_token with your new client id and secret to get an access token
6. Use that access token whenever you call a method
Authentication with client id and secret:
1. Call get_access_token with your new client id and secret to get an access token
2. Use that access token whenever you call a method
Note: The access token will eventually expire, so please call get_access_token again when it does
-
Scope | Scope Description |
---|---|
read:emotion | Get the emotion of text |
get_access_token
Code samples
# You can also use wget
curl -X POST https://api.sentimllc.com/token?client_id=string&client_secret=string \
-H 'Accept: application/json'
import sentim
configuration = sentim.Configuration()
# Defining host is optional and default to https://api.sentimllc.com
configuration.host = "https://api.sentimllc.com"
# Create an instance of the API class to request an api token
api_instance = sentim.DefaultApi(sentim.ApiClient(configuration))
path_to_credentials = "/path/to/credentials"
with open(path_to_credentials, "r") as f:
client_id, client_secret = f.readlines()[1].split(",")
# Configure OAuth2 access token for authorization: sentim_auth
configuration.access_token = api_instance.get_access_token(client_id, client_secret)
# override instance with access token now that we've authenticated
api_instance = sentim.DefaultApi(sentim.ApiClient(configuration))
POST /token
Oauth 2.0 authentication handler
Given your client id and secret (generated from the user settings page), generates the access token needed to authenticate with the rest of the api. Note: access tokens will eventually expire, so you will need to call this method periodically to get a new one.
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
client_id | query | string | true | Your client id |
client_secret | query | string | true | Your client secret |
Example responses
200 Response
"string"
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | Successful operation. Returns your access token. | string |
400 | Bad Request | Invalid client id or secret | None |
Response Schema
Chatbot Effectiveness API
score_chatbot_conversation
Code samples
# You can also use wget
curl -X POST https://api.sentimllc.com/chatbot_effectiveness/batch \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer {access-token}'
import sentim
api_instance = # ... see get_access_token to see how to get a proper instance
conv = sentim.Conversation([
"Hello!",
"Hello, how can I help you?",
"I'm having issues with the site.",
"I'm sorry to hear that. What is happening specifically?",
"After I click on the link provided, the webpage keeps saying it's not found.",
"Can you access google.com?",
"Why does that matter?",
"I just want to check if you are connected to the internet.",
"Yeah, I can access google.com",
"Okay, great! Can you access our home page?",
"Yes, I can access everything except the link that you sent me! AHHHHH!",
"Okay, I've sent you a new link. Can you check to see if the new link works?",
"Yea... one second. Yeap. The link works. Thanks!",
"Not a problem. Is there anything else I can help you with today?",
"Nope! Goodbye."
],
lang="Eng")
api_response = api_instance.score_chatbot_conversation(conv)
POST /chatbot_effectiveness/batch
Score the effectiveness of every chatbot message in a conversation
Given a conversation of user-chatbot speech, where the user is the first and last to talk, score every chatbot's message based on whether or not the user's emotion matches what we expect.
Body parameter
{
"ignore_first": true,
"lang": "en",
: [
,
]
}
Restrictions:
Input conversation must have at least three messages.
The number of messages must be of odd length (this is how we check if the conversation starts and ends with a message from the user).
The number of messages cannot exceed 25.
The length of each message cannot exceed 300.
Note: unlike in score_chatbot_effect, this method will still produce a score for text that exceeds the maximum length.
It will instead produce both an error result and a normal result indicating that it automatically truncated the text to the proper length and this was the result for the truncated text.
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
body | body | Conversation | true | none |
Example responses
200 Response
{
"ResultList": [
{
"Score": 0.70523544,
"Index": 1
},
{
"Score": 0.70523544,
"Index": 1
}
],
"ErrorList": [
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
},
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
}
]
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | Successful operation, returns the score for every chatbot message (i.e. every other message starting with the second message (2,4,6,8...)). | ConversationResponse |
400 | Bad Request | Invalid list value. Could be due to too many items in the list or having a list item too long. | None |
Response Schema
score_chatbot_effect
Code samples
# You can also use wget
curl -X POST https://api.sentimllc.com/chatbot_effectiveness/single \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer {access-token}'
import sentim
api_instance = # ... see get_access_token to see how to get a proper instance
conv = sentim.Conversation([
"Hello!",
"Hello, how can I help you?",
"I'd like to get a car.",
"What kind of car?",
"A truck."
],
lang="Eng")
api_response = api_instance.score_chatbot_effect(conv)
POST /chatbot_effectiveness/single
Score the effectiveness of the last chatbot message in a conversation
Given a conversation of user-chatbot speech, score the chatbot's response (the second to last message) based on whether or not the user's emotion matches what we expect.
Body parameter
{
"ignore_first": true,
"lang": "en",
: [
,
]
}
Restrictions:
Input conversation must have at least three messages.
The number of messages must be of odd length (this is how we check if the conversation starts and ends with a message from the user).
The number of messages cannot exceed 5.
The length of each message cannot exceed 300.
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
body | body | Conversation | true | none |
Example responses
200 Response
0
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | Successful operation. Returns the effectiveness score - (a number between 0 and 1, inclusive) | number |
400 | Bad Request | Invalid conversation. Conversation or messages too long? | None |
Response Schema
Emotion Recognition API
detect_batch_emotion
Code samples
# You can also use wget
curl -X POST https://api.sentimllc.com/emotion/batch \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer {access-token}'
import sentim
api_instance = # ... see get_access_token to see how to get a proper instance
textlist = [
'HELP me pls!',
"The world is on fire.",
"Everything sucks, and I hate it!"
] # list[str] | List of Text to classify
lang = 'Eng' # str | Language of the input text.
batch_text = sentim.BatchText(textlist, lang)
api_response = api_instance.detect_batch_emotion(batch_text=batch_text)
POST /emotion/batch
Detect the emotion of a list of strings
Given a list of strings and the language of all strings, this method produces the emotion scores for each input string. Note: This method assumes that all of the strings are unrelated to each other, i.e. this method does not use context.
Body parameter
{
"textlist": [
"Example 1",
"Example 2",
"Example 3",
],
"lang": "en"
}
Restrictions:
The number of documents cannot exceed 25.
The length of each document cannot exceed 300.
Note: This method does not automatically truncate text and will only return an error for items that exceed the document length.
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
body | body | BatchText | true | none |
Example responses
200 Response
{
"ResultList": [
{
"EmotionScore": {
"Anger": 0.70523544,
"Neutral": 0.00899112,
"Others": 0.3163906,
"Happy": 0.11595909,
"Joy": 0.02580458,
"Affection": 0.01137558,
"Sad": 0.00235727,
"Disappointment": 0.00223313
},
"Index": 0,
"Emotion": "Anger"
},
{
"EmotionScore": {
"Anger": 0.70523544,
"Neutral": 0.00899112,
"Others": 0.3163906,
"Happy": 0.11595909,
"Joy": 0.02580458,
"Affection": 0.01137558,
"Sad": 0.00235727,
"Disappointment": 0.00223313
},
"Index": 0,
"Emotion": "Anger"
}
],
"ErrorList": [
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
},
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
}
]
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | Successful operation. Returns the emotion for every string in the input. | BatchEmotionResponse |
400 | Bad Request | Invalid list or lang value. Could be due to too many items in the list or using an unsupported language. | Language of text. Must be one of these language codes. |
Response Schema
detect_emotion_conversation
Code samples
# You can also use wget
curl -X POST https://api.sentimllc.com/emotion/conversation \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer {access-token}'
import sentim
api_instance = # ... see get_access_token to see how to get a proper instance
conv = sentim.Conversation([
"Hello!",
"Hello, how can I help you?",
"I'm having issues with the site.",
"I'm sorry to hear that. What is happening specifically?",
"After I click on the link provided, the webpage keeps saying it's not found.",
"Can you access google.com?", "Why does that matter?",
"I just want to check if you are connected to the internet.",
"Yeah, I can access google.com",
"Okay, great! Can you access our home page?",
"Yes, I can access everything except the link that you sent me! AHHHHH!",
"Okay, I've sent you a new link. Can you check to see if the new link works?",
"Yea... one second. Yeap. The link works. Thanks!",
"Not a problem. Is there anything else I can help you with today?",
"Nope! Goodbye."
],
lang="Eng")
api_response = api_instance.detect_emotion_conversation(conv)
POST /emotion/conversation
Detect the emotion of every user message in a conversation
Given a conversation of user-chatbot speech, classify the emotion the user is expressing for every user message in the conversation.
Body parameter
{
"ignore_first": true,
"lang": "en",
: [
,
]
}
Restrictions:
If ignore_first is true, the input conversation must have at least 5 messages.
The number of messages must be of odd length (this is how we check if the conversation starts and ends with a message from the user).
The number of messages cannot exceed 25.
The length of each message cannot exceed 300.
Note: unlike in detect_emotion, this method will still produce a score for text that exceeds the maximum length.
It will instead produce both an error result and a normal result indicating that it automatically truncated the text to the proper length and this was the result for the truncated text.
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
body | body | Conversation | true | none |
Example responses
200 Response
{
"ResultList": [
{
"EmotionScore": {
"Anger": 0.70523544,
"Neutral": 0.00899112,
"Others": 0.3163906,
"Happy": 0.11595909,
"Joy": 0.02580458,
"Affection": 0.01137558,
"Sad": 0.00235727,
"Disappointment": 0.00223313
},
"Index": 0,
"Emotion": "Anger"
},
{
"EmotionScore": {
"Anger": 0.70523544,
"Neutral": 0.00899112,
"Others": 0.3163906,
"Happy": 0.11595909,
"Joy": 0.02580458,
"Affection": 0.01137558,
"Sad": 0.00235727,
"Disappointment": 0.00223313
},
"Index": 0,
"Emotion": "Anger"
}
],
"ErrorList": [
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
},
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
}
]
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | Successful operation. Returns the emotion for every user message (i.e. every other message starting with the first (1,3,5,...)) | BatchEmotionResponse |
400 | Bad Request | Invalid list or lang value. Could be due to too many items in the list or using an unsupported language. | Language of text. Must be one of these language codes. |
Response Schema
detect_emotion
Code samples
# You can also use wget
curl -X POST https://api.sentimllc.com/emotion/single \
-H 'Content-Type: application/json' \
-H 'Accept: application/json' \
-H 'Authorization: Bearer {access-token}'
import sentim
api_instance = # ... see get_access_token to see how to get a proper instance
conv = sentim.Conversation([
"Hello!",
"Hello, how can I help you?",
"I'd like to get a car.",
"What kind of car?",
"A truck."
],
lang="Eng")
api_response = api_instance.detect_emotion(conv)
POST /emotion/single
Detect emotion of a conversation
Given a conversation and the language of that conversation, this method classifies the emotion expressed for the last message of the input conversation.
Body parameter
{
"ignore_first": true,
"lang": "en",
: [
,
]
}
Restrictions:
Input conversation must have between 1 and 5 messages.
The number of messages must be of odd length (this is how we check if the conversation starts and ends with a message from the user).
The length of each message cannot exceed 300.
Parameters
Name | In | Type | Required | Description |
---|---|---|---|---|
body | body | Conversation | true | A conversation of length 1-5 turns. |
Example responses
200 Response
{
"EmotionScore": {
"Anger": 0.70523544,
"Neutral": 0.00899112,
"Others": 0.3163906,
"Happy": 0.11595909,
"Joy": 0.02580458,
"Affection": 0.01137558,
"Sad": 0.00235727,
"Disappointment": 0.00223313
},
"Emotion": "Anger"
}
Responses
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | Successful operation. Returns the emotion of the last message in the conversation. | EmotionResponse |
400 | Bad Request | Invalid text or lang value. Text size limit exceeded? | Language of text. Must be one of these language codes. |
Response Schema
Schemas
BatchEmotionResponse
{
"ResultList": [
{
"EmotionScore": {
"Anger": 0.70523544,
"Neutral": 0.00899112,
"Others": 0.3163906,
"Happy": 0.11595909,
"Joy": 0.02580458,
"Affection": 0.01137558,
"Sad": 0.00235727,
"Disappointment": 0.00223313
},
"Index": 0,
"Emotion": "Anger"
},
{
"EmotionScore": {
"Anger": 0.70523544,
"Neutral": 0.00899112,
"Others": 0.3163906,
"Happy": 0.11595909,
"Joy": 0.02580458,
"Affection": 0.01137558,
"Sad": 0.00235727,
"Disappointment": 0.00223313
},
"Index": 0,
"Emotion": "Anger"
}
],
"ErrorList": [
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
},
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
}
]
}
Properties
Name | Type | Required | Description |
---|---|---|---|
ErrorList | [ErrorItem] | false | The list of errors that occured during the execution of this method. |
ResultList | [ResultItem] | false | The list of results. |
Conversation
{
"ignore_first": true,
"lang": "en",
: [
,
]
}
Properties
Name | Type | Required | Description |
---|---|---|---|
conversation | [string] | true | Conversation as a list of alternating messages, starting with the user. |
lang | string | true | Language spoken in the conversation as a language code. Must be one of these language codes. |
ignore_first | boolean | false | If true, only the last chatbot or user message will be scored. Primarily used for stitching together long conversations that exceed the max conversation length. Default: false. |
ConversationScore
{
"Score": 0.70523544,
"Index": 1
}
Properties
Name | Type | Required | Description |
---|---|---|---|
Index | integer | false | The index of the message in the conversation that the score is for. |
Score | number | false | The score, between 0 and 1, representing how well the user's response to this message matches what we would expect. A lower score indicates that the user's emotional state is worse than we expected, so the message probably need modified. |
ConversationResponse
{
"ResultList": [
{
"Score": 0.70523544,
"Index": 1
},
{
"Score": 0.70523544,
"Index": 1
}
],
"ErrorList": [
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
},
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
}
]
}
Properties
Name | Type | Required | Description |
---|---|---|---|
ErrorList | [ErrorItem] | false | The list of errors that occured during the execution of this method. |
ResultList | [ConversationScore] | false | The list of index and effective score for every chatbot message. |
EmotionScore
{
"Anger": 0.51688863,
"Neutral": 0.00899112,
"Others": 0.3163906,
"Happy": 0.11595909,
"Joy": 0.02580458,
"Affection": 0.01137558,
"Sad": 0.00235727,
"Disappointment": 0.00223313
}
The probability of each emotion in the message. The total probability of all emotions must sum to one, and each emotion's probability is between 0 and 1.
Properties
Name | Type | Required | Description |
---|---|---|---|
Anger | number | false | none |
Disappointment | number | false | none |
Sad | number | false | none |
Happy | number | false | none |
Joy | number | false | none |
Affection | number | false | none |
Neutral | number | false | none |
Others | number | false | none |
EmotionResponse
{
"EmotionScore": {
"Anger": 0.70523544,
"Neutral": 0.00899112,
"Others": 0.3163906,
"Happy": 0.11595909,
"Joy": 0.02580458,
"Affection": 0.01137558,
"Sad": 0.00235727,
"Disappointment": 0.00223313
},
"Emotion": "Anger"
}
Properties
Name | Type | Required | Description |
---|---|---|---|
Emotion | string | false | The emotion with the highest likelihood in the emotion score. |
EmotionScore | EmotionScore | false | The probability of each emotion in the message. The total probability of all emotions must sum to one, and each emotion's probability is between 0 and 1. |
ErrorItem
{
"Index": 0,
"ErrorCode": 6,
"ErrorMessage": "ErrorMessage"
}
Properties
Name | Type | Required | Description |
---|---|---|---|
Index | integer | false | The index of the conversation or list that the error occurred. |
ErrorMessage | string | false | The message describing what went wrong. |
ErrorCode | integer | false | The error code of the message. Usually one of https://en.wikipedia.org/wiki/List_of_HTTP_status_codes. |
ResultItem
{
"EmotionScore": {
"Anger": 0.70523544,
"Neutral": 0.00899112,
"Others": 0.3163906,
"Happy": 0.11595909,
"Joy": 0.02580458,
"Affection": 0.01137558,
"Sad": 0.00235727,
"Disappointment": 0.00223313
},
"Index": 0,
"Emotion": "Anger"
}
Properties
Name | Type | Required | Description |
---|---|---|---|
Index | integer | false | The index of the conversation or list that was classified. |
Emotion | string | false | The classified emotion of the message. |
EmotionScore | EmotionScore | false | The probability of each emotion in the message. The total probability of all emotions must sum to one, and each emotion's probability is between 0 and 1. |
Text
{
"text": "string",
"lang": "en"
}
Properties
Name | Type | Required | Description |
---|---|---|---|
text | string | true | Text to classify |
lang | string | true | Language of the input text. Must be one of these language codes. |
BatchText
{
"textlist": [
"Example 1",
"Example 2",
"Example 3",
],
"lang": "en"
}
Properties
Name | Type | Required | Description |
---|---|---|---|
textlist | [string] | true | List of Text to classify |
lang | string | true | Language of the input text. Must be one of these language codes. |
General Errors
Error Code | Error Message | Error Description |
---|---|---|
401 | Invalid access token. | Access token is invalid or has expired. Please check to see if you are getting the access token correctly or get a new access token. |
402 | Invalid payment option. | Your credit card information provided from the user settings page is incorrect. Perhaps your card has since been canceled or has expired. Please go to your user settings and click the "change credit card info" button to update your credit card information securely. |